Advanced Data-Driven Testing with Selenium Java
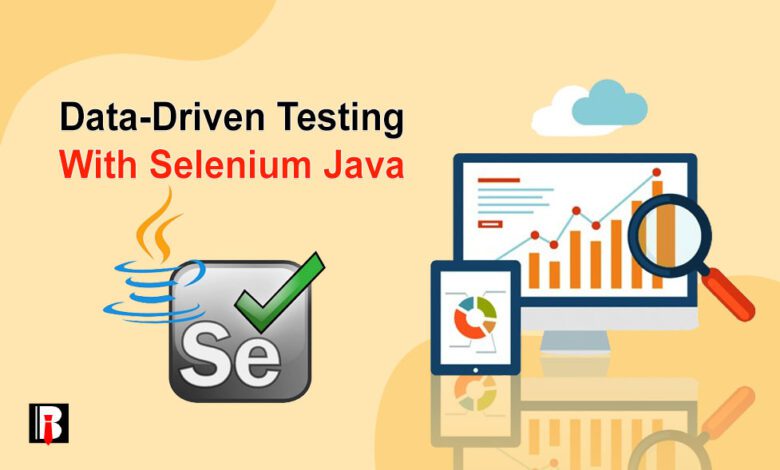
Are you a beginner developer looking to incorporate data-driven testing using Selenium Java? This article aims to guide you through data-driven testing methods that can help you maintain the quality and dependability of your applications.
Selenium is widely recognized as a contender in automated web application testing thanks to its adaptability, versatility, and support for programming languages. Conversely, data-driven testing is favored for its capability to execute test scripts with datasets thereby enhancing test coverage and efficiency when used with Selenium Java.
Quick Shortcut Headlines
Exploring Data-Driven Testing
To cover the basics, we can say that data-driven testing is a modern methodology where the test data will be completely stored separately from the test scripts. By adopting this approach, the testers can use a single test script to execute multiple test instances with different sets of data. To further improve our knowledge of this process, we have stated some of the major advantages of this process:
- It helps improve reusability by using one script to test multiple data scenarios.
- Using data-driven testing, app developers can massively improve the maintainability of test instances, as test data changes will not require the implementation of changes to the entire test cycle.
- Since the test data is separated from the test case, the developers can easily add more test cases by adding new data sets, which can help improve the test instances’ scalability.
Setting up the Environment
Before we start understanding the implementation of data-driven testing with Selenium Java, let us understand how the developers can create the test environment for this implementation. For this process, the app developers have to first ensure that they have installed the following dependencies:
- Downloading the Java development kit version 8 or later from the official website is important.
- After this, the developers have to download and integrate the development environment of their choice. Some of the popular options include Eclipse and IntelliJ IDEA.
- The developers must also install Maven to manage the dependencies and execute the test instances.
- Finally, it is important to integrate Selenium WebDriver as a dependency within the ‘pom.xml’ file. This dependency will be responsible for executing all the test instances in the native environment of your target browsers.
Maven Dependencies
The developers must also include a series of dependencies in the ‘pom.XML’ file, including Selenium and Apache POI. These integrations are also crucial for Excel handling. To perform this integration, testers simply have to enter the following code snippet in the terminal window:
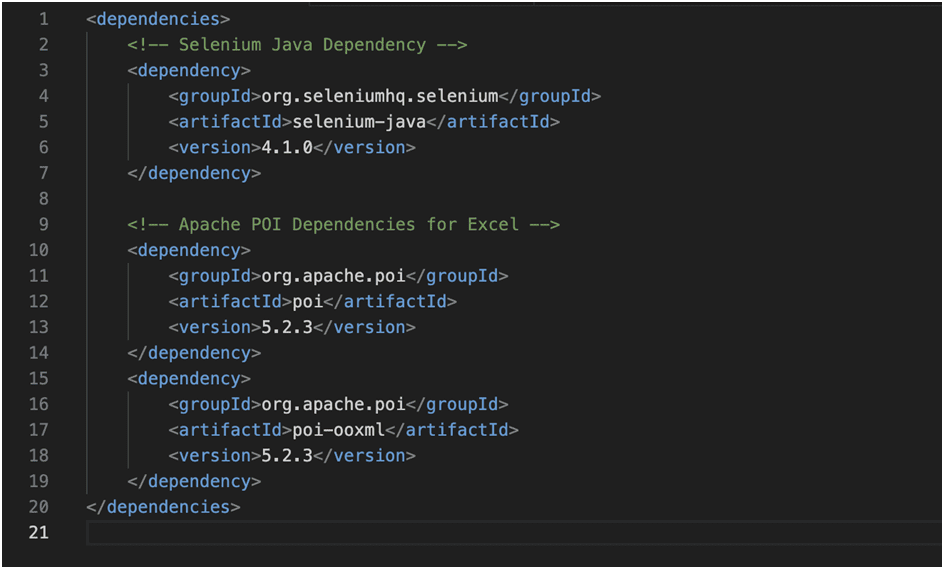
Implementing Data-Driven Testing with Selenium Java
The application developers and testers must follow a series of steps to implement data-driven testing with Selenium Java. To simplify this knowledge for the new developers and testers, we have mentioned all of these steps in the intended chronological order:
1. Creating Test Data
To better understand this example, we will use an Excel file for reference that will help the testers store the test data. To start this process, the testers must create an Excel file named ‘TestData.xlsx.’ This test data will contain the username, password, and expected result columns.
2. Reading Excel Data With Apache POI
Now that you have created the test data, you must create a utility class to read the data from the Excel file. To create this class, the application tester simply has to enter the following code snippet in the terminal window:
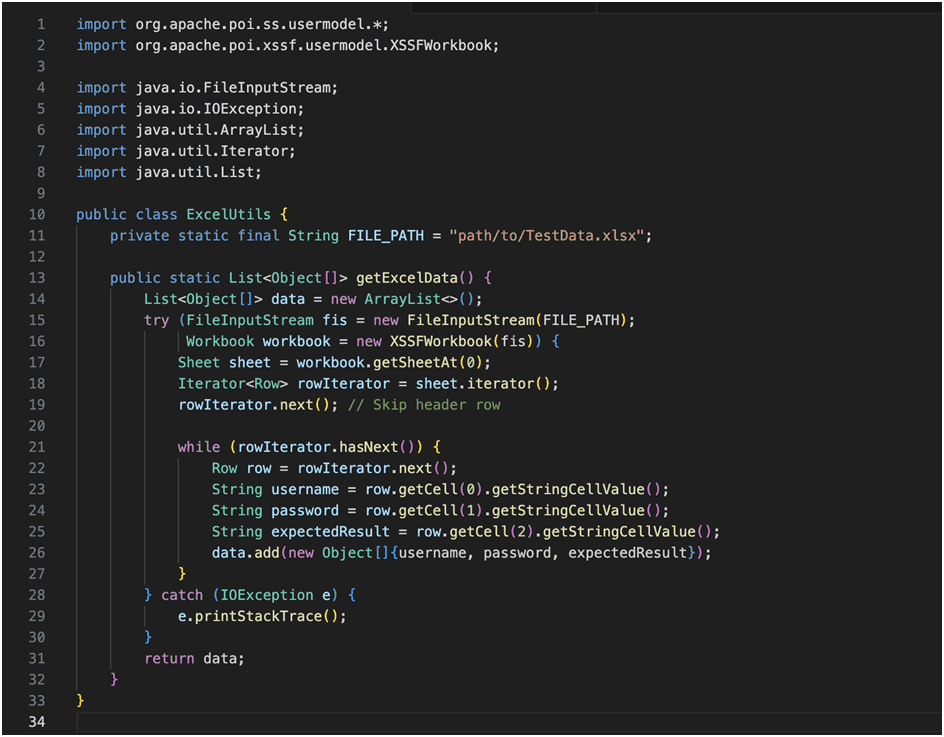
3. Writing the Selenium Test
It is time for the testers to create a test class using TestNG. After creating this class, the application testers must integrate the data with the Excel separately. We have mentioned this sample code snippet that will help the testers perform this process:
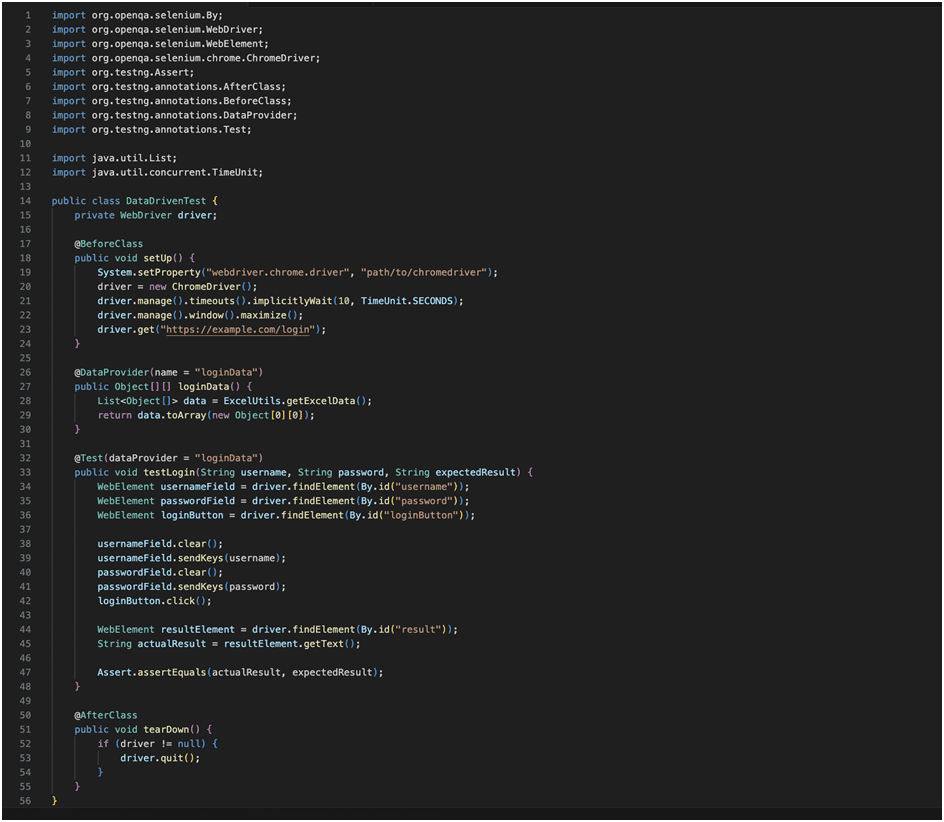
4. Running the Test Cases
The final step in this process is to execute the test cases using the integrated development environment or the command line. TestNG will execute the ‘testLogin’ method multiple times during this process. These iterations will use different data sets from the Excel file we integrated in the previous step.
Advanced Techniques for Data-Driven Testing
After implementing data-driven testing with Selenium Java, there are certain factors that you must consider that will have a crucial role in improving the productivity and efficiency of the test cases. To enlighten the new testers about this segment, we have mentioned some of the most important additions to this list:
1. Parameterizing Data from Multiple Sources
The testers can extend data-driven testing to access data from multiple sources, including CSV files, databases, and APIs. By using parameterized data sources for the test execution process, the application developers can further improve the test accuracy by integrating various real-world parameters into the test source.
To implement this process, the testers must create separate utility classes for handling different data sources and integrate them with the test instances.
2. Parallel Test Execution
A glance at the present testing cycles will reveal that the developers have to verify the proper functioning of thousands of app infrastructure elements. So, to improve the efficiency of these test instances, the developers can integrate parallel testing with the automation test instances.
To implement this process, the testers must update their ‘testing.xml’ file and include the required configuration. To further simplify this process, we mentioned the code snippet to help the developers get started.
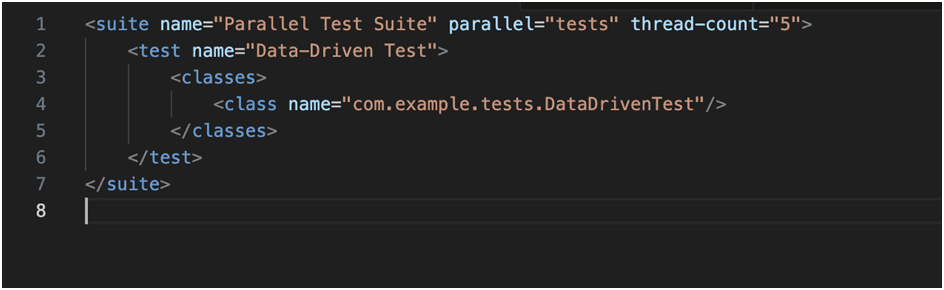
3. Using Real Device Testing
To further improve the efficiency of data-driven testing with Selenium, we highly recommend that testers integrate real device testing. Using this process, testers can analyze the apps’ performance when subjected to various physical devices.
To avoid the expense and hassle of setting up and maintaining an in-house device testing lab, the application developers can integrate various online device farms that are easily available in the market. This device farm provides access to real devices through various remote servers.
One such cloud-based platform is LambdaTest, that can execute data-driven test instances on more than 3000+ browsers and OS combinations. It massively benefits the test data management and execution process.
This platform also helps improve the test tracking and debugging process using real-time test activity logging and comprehensive test report generation.
4. Using Page Object Models (POMs)
The Page Object Model is a modern design pattern that enhances the maintainability of the test scripts by encapsulating the web elements and their interactions within separate classes. Using this process, the application developers can also massively boost the readability of the test cases as it removes the technical aspects into a separate entity.
By integrating the following code snippet into the terminal window, the application developers will be able to integrate page object models while executing data-driven testing with Selenium Java:
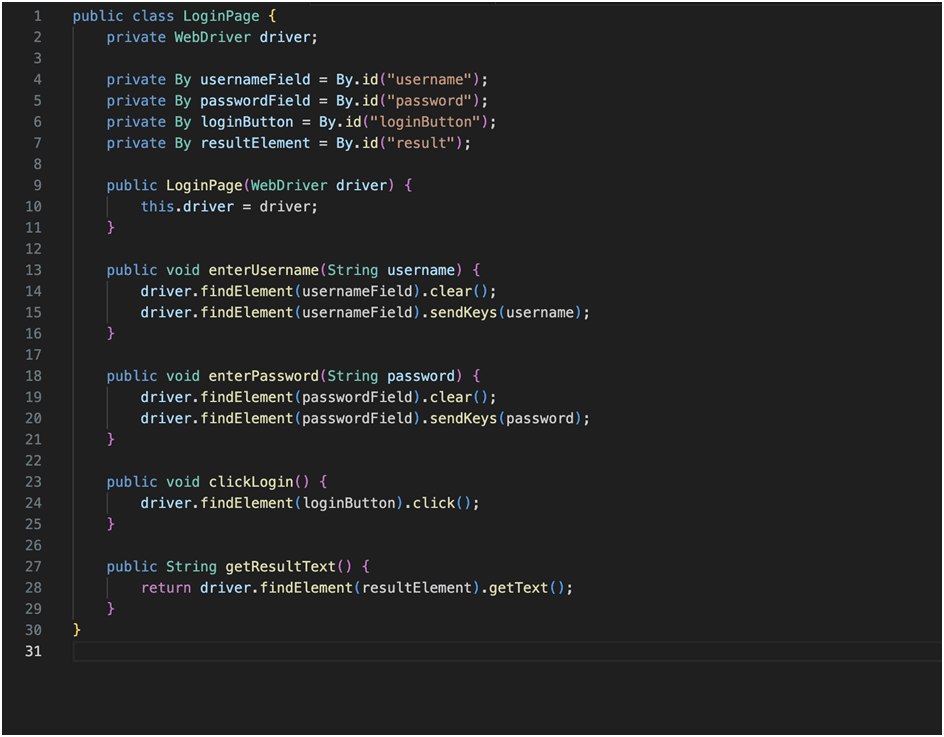
5. Error Handling and Logging
We would advise the testers to utilize robust error classes and logging mechanisms, as they are very important for diagnosing issues and ensuring the reliability of the test instances. It is also important to integrate logging frameworks like log4J to capture detailed logs during the test execution process.
To add log4J dependency within the ‘pom.xml’ file, the application testers have to integrate the following code snippet in the terminal window of Selenium:
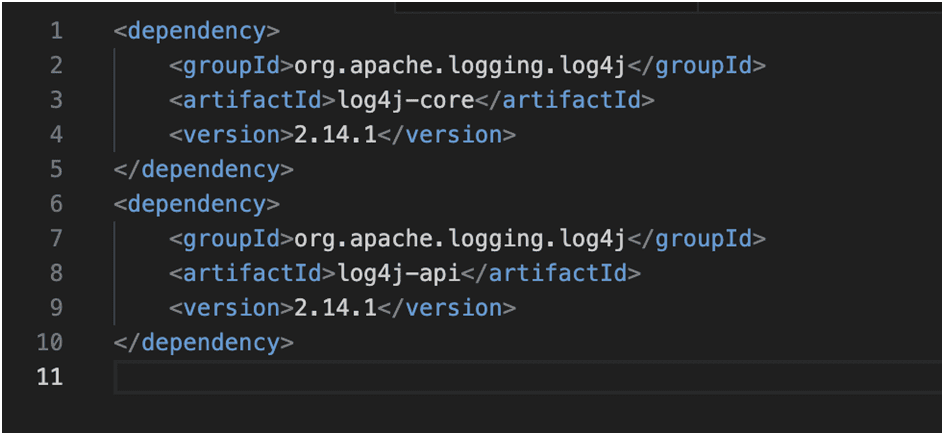
After this process, the application testers must enter the following code snippet to create a configuration file named ‘log4j2.xml.’
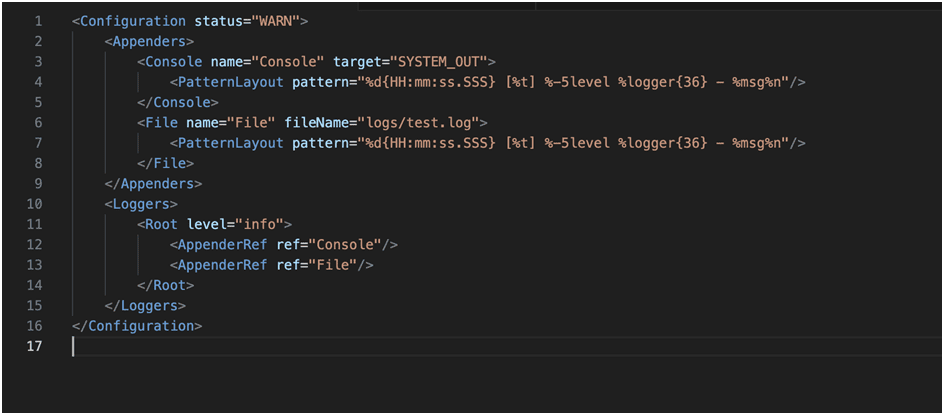
Finally, the following code snippet will allow the developers to integrate log4J with the data-driven test instances and run them during the test execution.
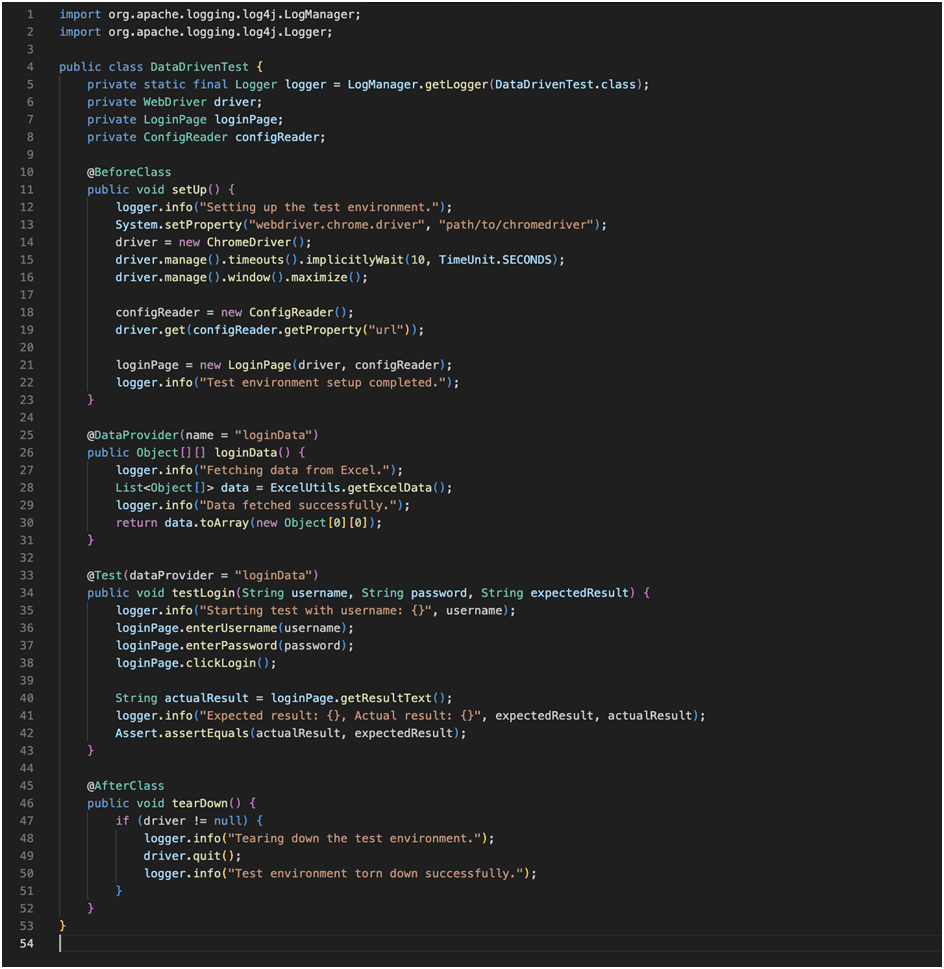
Best Practices for Running Data-Driven Testing with Selenium Java
Let us now divert our attention to some of the best practices the developers and testers must use within the test environment to streamline the productivity of the test instances:
- While downloading all the prerequisite files and software for this process, we highly recommend the developers use the official websites to avoid any details or possibilities of malicious attacks.
- Before the new testers start to adopt Selenium, it is very important to review the official documentation on the website. This documentation will explain the functioning of all the methods using sample test cases. It also provides details of some of the most common issues that can arise in the testing process with their solutions.
- Since Selenium is an open-source test automation suite, it has a massive community of enthusiastic developers and testers. So, new testers can refer to this community whenever they are stuck with any Selenium feature.
- While executing parallel test instances, we advise the testers to adopt a standard vocabulary that names the test cases according to the target elements. This practice helps avoid confusion or data mix-ups when developers work with thousands of test incidences.
- Before beginning data-driven testing with Selenium Java, the testers must create a comprehensive test plan to store information regarding all the tools, testing strategies, and possible resolutions. This plan will also act as a source to track the current status of the application development project for all the company members.
- Finally, the app developers must maintain standard documentation to keep track of all the testing results, bugs detected, and the resolutions implemented. This will help avoid similar errors in future application iterations and seamlessly roll out future updates.
The Conclusion
Ultimately, we can say that advanced data-driven testing with Selenium and Java will provide a robust framework for ensuring the reliability and quality of modern web apps. By implementing all the techniques we discussed in this article and incorporating the best practices, the testers can create maintainable and scalable test suits.
We would also like to highlight that all the techniques covered in this article are designed to help the new testers get started with advanced data-driven testing. However, it is also important to remember that there is always room for further enhancement and optimization.
So, we would advise the testers to constantly refine their approach to ensure the tests remain efficient and effective with the app’s evolution.