Advanced Mocking Techniques with JUnit for Agile Development
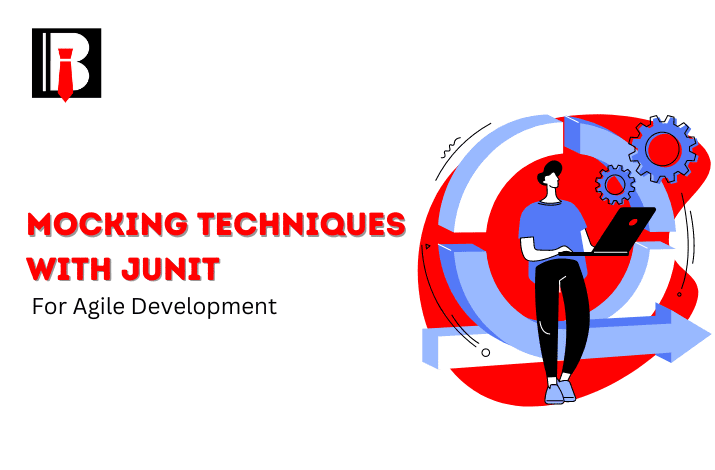
Are you a new tester struggling to implement mocking techniques with JUnit testing?
Mocking techniques while performing JUnit testing allow the testers to isolate the code under test and simulate various scenarios to analyze its behavior. In this segment, JUnit is a popular name for testing frameworks that run on Java. It also works very well with various mocking frameworks like Mockito to help in advanced testing strategies.
In this article, we will explain the advanced mocking techniques for JUnit testing in detail. We will also demonstrate how the testers can use these techniques to enhance the robustness and efficiency of unit test cases during the agile development process.
Quick Shortcut Headlines
What is Mocking
To quickly develop a basic idea, we can say that mocking is the process of creating elements that stimulate the behavior of real elements. Testers use these objects to isolate the unit of a code being tested. The testers can perform this process by mimicking the behavior of dependencies accordingly.
Mocking has become a very important parameter of the modern app creation industry as developers have to serve a versatile range of end users with different preferences, device choices, and expectations for the app. It also helps prevent the possibility of a failure when it is subjected to real-world conditions like user errors or overloaded traffic.
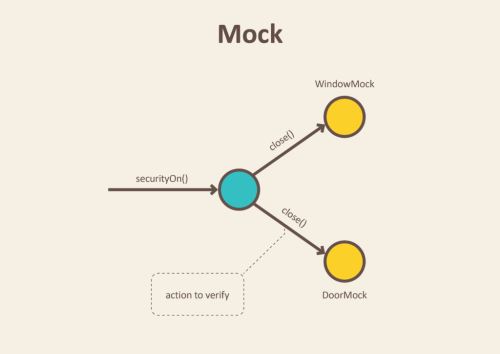
Importance of Mocking in Agile Development
While implementing Agile Development, the developers need quick iterations and frequent testing cycles. In such a scenario, mocking will help the application developers and testers in the following ways:
- It will help to isolate the code that is currently being interested and verify its performance based on multiple real-world parameters.
- Using this technique, the application developers can simulate complex or unavailable dependencies to further improve the scope and scalability of the testing infrastructure.
- It is one of the best solutions for testing error scenarios and edge cases.
- Using mocking in agile development, the application testers can massively speed up the testing process so they can perform this step by avoiding slow operations, including network calls.
What is JUnit
For the sake of the new testers, we will take a basic look at JUnit. At a base level, it is an open-source framework that is mainly designed to help with the action of writing and executing test cases with Java. JUnit also provides a simple yet powerful way of defining the test cases to organize the test code and execute the final test instances.
To further improve our knowledge about JUnit and its scope in the modern testing infrastructure, we have mentioned some of its most important aspects:
- JUnit uses annotations to mark methods as test methods, configure the test setups, and tear down processes. Some common methods of JUnit are @Test, @Before, and @After.
- Assertions are important as JUnit will use these to check the expected outcomes during the test execution process. These methods include assertEquals, assertTrue, and assertFalse.
- JUnit uses test runners to execute the actual test instances. The default test runner of JUnit runs the test method annotations with @Test.
- Integrating JUnit with various integrated development environments like IntelliJ IDEA and Eclipse is a very simple and easy process. The developers can also easily integrate JUnit with various build tools like Gradle and Maven. Finally, JUnit natively supports various continuous integration servers like Jenkins.
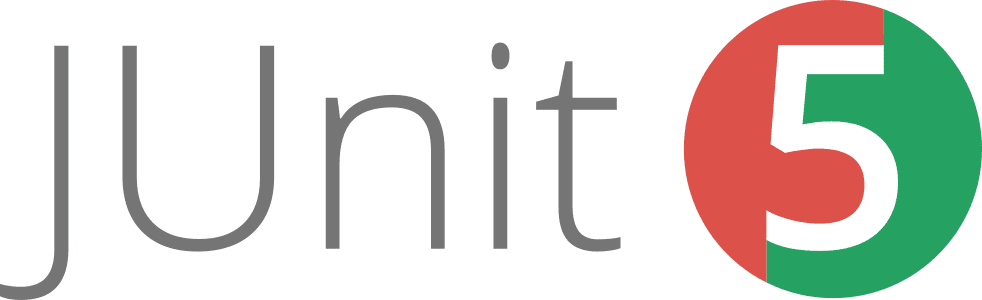
Setting up the Environment
Before understanding the advanced techniques to implement mocking while using Agile development with JUnit, the application testers must create an idea about setting up the basic test environment. To shed more light on these segments, we have mentioned all the required steps in the intended order:
Read Also: Benefits Of Progressive Web Apps For Ecommerce
JUnit Setup
JUnit is often called the backbone of executing unit testing while using Java. So, to start with the JUnit setup process, the application testers must include a series of dependencies in the ‘pom.xml’ file. However, it is worth mentioning that this file is only usable for the testers using Maven.
For JUnit 4 users, you must enter the following code in the terminal window to integrate the dependencies.
If you are a JUnit 5 user, you will have to enter the following code snippet in the terminal window to complete the above process:
Setting Up Mockito
Now that we have set up JUnit, it is time to set up the mocking environment using Mockito. For a basic introduction, we can say that Mockito is a powerful and easy-to-use mocking framework. The setup process is pretty simple, as you simply have to enter the following code snippet in the terminal window to add it as a dependency:
Basic Mocking With Mockito
Before moving on to the advanced mocking techniques, let us cover the basic ones to create a base-level understanding for the new developers and testers:
- The first step in this process involves creating the mocks. So, to perform this process, the testers must use the ‘Mockito. mock()’ method. To implement this method, the application testers must simply enter the following command in Mockito’s terminal window.
- The first method that we are going to discuss is the stubbing method. It defines what a mock should return when the system calls its method. To implement this method, the application testers simply have to enter the following command in the terminal window:
- The next method deals with verifying the interactions with the elements of the application. Mockito allows the testers to verify the interactions with mocks to ensure they are called with specific arguments. To implement this method, the testers simply have to enter the following command in the terminal window:
Advanced Mocking Techniques for JUnit Testing
Now that we have covered all the basic techniques for implementing mocking with JUnit let us go through some of the most advanced techniques that can further elevate the scope and quality of the test instances:
- Mocking Static Methods
Mocking the static methods using the standard Mockito interface is often challenging. However, developers can use PowerMockito, which extends Mockito’s capabilities and allows it to handle static methods. To integrate this new method as a dependency, the application testers simply have to enter the following command in the terminal window:
We have also mentioned a sample code snippet that demonstrates the mocking mechanism of a static method using power Mockito:
- Mocking Final Classes and Methods
PowerMockito also allows the developers to mock final classes and methods. These assets are normally unmockable in a traditional testing environment due to various restrictions.
We have also mentioned a sample code snippet that demonstrates how the testers can mock classes and methods using the above-mentioned approach:
- Using Argument Captors
Using argument captures, the testers can capture the arguments passed to mocked methods for further assertions. In simple terms, it acts as an extra verification layer to ensure that all the test cases are accurate and properly evaluate the performance of the elements on the application.
By entering the following command in the terminal window, the application developers can easily initiate this process:
- Mocking With Answer
The developers can use the “Answer” interface to define custom behavior when the system calls methods during the execution process. By entering the following command snippet in the terminal window, the developers can initiate this process:
- Mocking Private Methods
The developers will also have to use PowerMockito if they are trying to implement the mocking process of private methods. We have mentioned a sample code snippet that can allow the developers to perform this process:
Integration with JUnit
It is worth mentioning that JUnit 5 introduces a more flexible extension model that replaces JUnit 4’s runners and rules.
The following code snippet shows the execution of the Mockito rule with JUnit 4:
Now, the following code shows the execution of the same process with JUnit 5 and Mockito extension:
Best Practices for Mocking With JUnit Testing
Finally, we are going to discuss some of the best practices that can help the Java testers to enhance their productivity while implementing mocking with JUnit testing:
- The application developers need to keep the test cases maintainable by using clear and descriptive names for test methods. They should also arrange the test cases using the AAA pattern, which stands for Arrange, Act, and Assert. It is also important to ensure the test cases focus on a single behavior simultaneously.
- We advise the application testers to balance the mocking and realism aspects of the test execution process. They can perform this by avoiding over-mocking, which can lead to brittle test instances. It is also important to prefer real objects for simple dependencies to keep the test cases realistic overall.
- During the entire mocking process, the app tester’s primary focus should be on the performance of the application and the testing phase as a whole. To implement these, the testers can use mocks to speed up test cases, which avoids slow operations. It is also important to ensure the mock setup and verification process are not overly complex, which can slow down the test execution phase.
- To further improve the accuracy of test instances, the developers can integrate remote real device farms with cloud platforms like LambdaTest. It is an AI-powered online device farm and test execution platform that allows you to run manual and automated tests at scale. It enables you to perform cross-browser testing with over 3000+ real devices, browsers, and OS combinations.
The Bottom Line
Based on all the factors we analyzed in this article, advanced mocking techniques with JUnit and Mockito will provide powerful tools for writing robust and maintainable unit test cases. Moreover, these techniques will be important for agile development, where reliable and rapid testing is the backbone of the entire process.
The testers must master these techniques to ensure the code is well-tested, leading to high-quality software and faster delivery times. Finally, the testers must realize that mocking is not only about creating test doubles but also enhances test efficiency from clarity and robustness.
By using all the advanced techniques discussed in this article, testers can ensure that the test cases are comprehensive and maintainable, ultimately contributing to a smoother and more effective agile development process.